
| 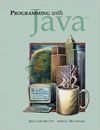 |
Table of ContentsProgramming in Java
Chapter 1-Introducing Java
Java
OOP
Creating Your First Applet
Running the Applet
Finding and Fixing Errors
Changing Fonts and Colors
Using HTML to Run the Applet in a Browser
Planning a Project
Your Hands-on Programming Example
Chapter 2-Using Variables and Constants
Classes and Methods
Variables and Constants
Text Components
System Dates
Buttons
Mouse Events
Your Hands-on Programming Example
Chapter 3-Designing the Interface with Layout Managers
Java Layout Managers
Your Hands-on Programming Example
Chapter 4-Performing Calculations and Formatting Numbers
Calculation Operators
Converting between Data Types
Formatting Numeric Output
Handling Exceptions
Using the Wrapper Data Classes
Your Hands-on Programming Example
Chapter 5-Creating Classes
Review of Object-Oriented Programming
Creating a New Class
Public vs. Private
Returning Values from a Method
Passing Arguments to a Method
Dividing an Applet Class
Adding a Constructor
Obtaining Values from Private Class Variables
Using a Class Variable for a Total
Creating a Class for Formatting
Your Hands-On Programming Example
Chapter 6-Decisions and Conditions
Decision Statements
Conditions
Nested If Statements
The Conditional Operator
Validating User Input
Programming for Multiple Button Objects
Precedence of Assignment, Logical, and Relational Operators
Your Hands-on Programming Example
Chapter 7-Making Selections with Check Boxes and Option Buttons
Check Boxes and Option Buttons
The switch Statement
Swing Components
Your Hands-on Programming Example
Chapter 8-Using Lists, Choices, and Looping
Lists
The Choice Class
Loops
Swing Lists
Your Hands-on Programming Example
Chapter 9-Arrays
Arrays
Using Array Elements for Accumulators
Table Lookup
Multidimensional Arrays
Lookup Operation for Two-Dimensional Tables
Creating an Array of Objects
Java Arrays for C++ and Visual Basic Programmers
Your Hands-on Programming Example
Chapter 10-Applications, Frames, Menus, and Dialogs
Applications
Frames
Menus
Dialogs
Multiple Frames
Popup Menus
A Swing Application
Running as an Application or an Applet
Your Hands-on Programming Example
Chapter 11-Multimedia in Java: Images, Sounds, Animation, and Video
Graphics
Using Image Files
Sound
Using the Graphics Object to Print
Animation
Swing Components
Your Hands-on Programming Example
Chapter 12-More OOP, Interfaces, and Inner Classes
OOP Review
Inheriting from your own classes
Interfaces
Inner Classes
Your Hands-on Programming Example
Chapter 13-Storing Information, Object Serialization, and JDBC
Streams
DataBase Connection with the JDBC API
SQL
Updating a Database
Your Hands-on Programming Example
Chapter 14-JavaScript
Scripting
Creating Your First JavaScript Program
The Object Model
Functions
Variables
Control Structures
Fun with JavaScript-Image Rollovers
Chapter 15-Advanced Features of Java
Software Development using Components
Client/Server Applications
Internationalization
Accessibility
Security
Your Hands-on Programming Example
Appendix A-Using an IDE
Appendix B-Conventions and Standards
Appendix C-Java 1.0 Event Handling and Deprecated Methods
Appendix D-Solutions to Feedback Questions
Appendix E-Creating JAR Files
Appendix F-Math Functions
Appendix F-Debugging |
|
|